
Core Features
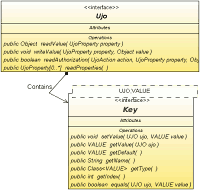
- object architecture based on two interfaces
- very simple introspection
- serialization to / from formats XML, CSV and Resource bundle
- unique object-relation mapping (ORM) solution is available for mapping to RDMBS
- special validators can be located into ujo-keys to check a consistency of the ujo-bean data
- UJO object controls an access to its key for different actions
- JavaBeans ready
- it is easy to clone, copy, sort and compare
- direct support for the JTable
- way for an implementation a listener to only one place for each keys including children
- unlike some competing projects the Key instance is immutable (hence never contains value) and the encapsulation is supported
- most features are checked by unit tests
- tiny framework without further dependencies

Architecture
- Key.setValue(Ujo, VALUE) - to write the value into the object and
- Key.getValue(Ujo) - to read the value.
- AbstractUjo - the great performance implemented by the object array
- MapUjo - a memory safe solution for the case of the sparse attribute values, the solution is based on the object HashMap
- BeanUjo - implementation calls related methods of JavaBean using a Java reflection

Sample of usage:
import org.ujorm.core.*; public class Person extends AbstractUjo { private static final KeyFactory f = newFactory(Person.class); public static final Key<Person,String > NAME = f.newKey(); public static final Key<Person,Boolean> MALE = f.newKey(); public static final Key<Person,Double > CASH = f.newKey(); @Override public KeyList<?> readKeys() { return f.getKeys(); } }
Note that a result of the method get(CASH) needs no casting to Double type and the Java compiler disallows improper parameter types in the statement set(CASH, newCash). The CASH key have got a default zero value to all Person instances similar like a primitive type do it.
The sample uses an extended API for more conventional key access in a source code that's available since the UJO release 0.80. The new solution allows to you to chain more keys according to a model of a some new popular languages. The extended API is a child of the original API so you can mix both types because all internal tools are handling the original API still .
Sometimes it is not possible to extend an abstract class so you can implements the Ujo interface by four methods only. Note the implementation is valid also for all subclasses of the Person class. The simple example:
import org.ujorm.*; import org.ujorm.core.*; public class Person implements Ujo { private static final KeyFactory<Person> f = KeyFactory.CamelBuilder.get(Person.class); public static final Key<Person,String > NAME = f.newKey(); public static final Key<Person,Boolean> MALE = f.newKey(); public static final Key<Person,Double > CASH = f.newKeyDefault(0.0); // --- The start of the Ujo implementation --- private Object[] data = new Object[f.lockAndSize()]; public Object readValue(Key key) { return data[key.getIndex()]; } public void writeValue(Key key, Object value) { data[key.getIndex()] = value; } public KeyList<?> readKeys() { return f.getKeys(); } public boolean readAuthorization(UjoAction action, Key key, Object value) { return true; } // --- The end of the Ujo implementation --- /** Sample method, how to add a new cash */ public void addCash(double cash) { double newPrice = CASH.of(this) + cash; CASH.setValue(this, newPrice); } }
Compare the both samples with a sample code of a JavaBean object. If we exclude all imports from the code then the Ujo implementation have got slightly less code lines in compare to JavaBeans. However the more keys gives significantly the better effect in favour of the Ujo implementation.
Reference projects

- persistence is made by Ujorm Core including application parameters.
- all table model are a child of UjoTable class.
- very small size: 200 kB (signed) including the Ujorm Core library.
- the jWorkSheet have used features of UJO release 0.7x (hard core).
You can see some ORM applications there.

Licence:
Copyright 2007-2022 Pavel Ponec
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.

Download:
<dependency> <groupId>org.ujorm</groupId> <artifactId>ujo-core</artifactId> <version>${ujorm.version}</version> </dependency>and the next extended dependency for the ORM:
<dependency> <groupId>org.ujorm</groupId> <artifactId>ujo-orm</artifactId> <version>${ujorm.version}</version> </dependency>Get the latest version of the source code by the git statement:

Similar projects:
- Joda-Beans - is a framework that radically enhances working with beans. The aim is to add type-safety, refactorability and compiler-checking to keys on beans.
- Bean-keys - project using Java 5 syntax and object oriented features in order to provide key support that leapfrogs the keys available in Delphi/VB and other languages. The keys implemented in bean-keys use Java's abilities (interfaces, annotations, generics etc.) to create a more powerful, extensible and object oriented system to expose component state.
- Commom Attributes - an attribute and metadata solution for the Java 1.4 or earlier enables Java programmers to use C#/.Net-style attributes in their code
- JSR-175 - a metadata facility for the JavaTM Programming Language
- MetaClass - the attributes are usually specified in the java source files as javadoc markup. The source file is then processed and the metadata is compiled into descriptors that MetaClass is capable of reading. These descriptors are then loaded at runtime to provide metadata about the program elements.
- qDox - a high speed, small footprint parser for extracting class/interface/method definitions from source files complete with JavaDoc @tags. It is designed to be used by active code generators or documentation tools.